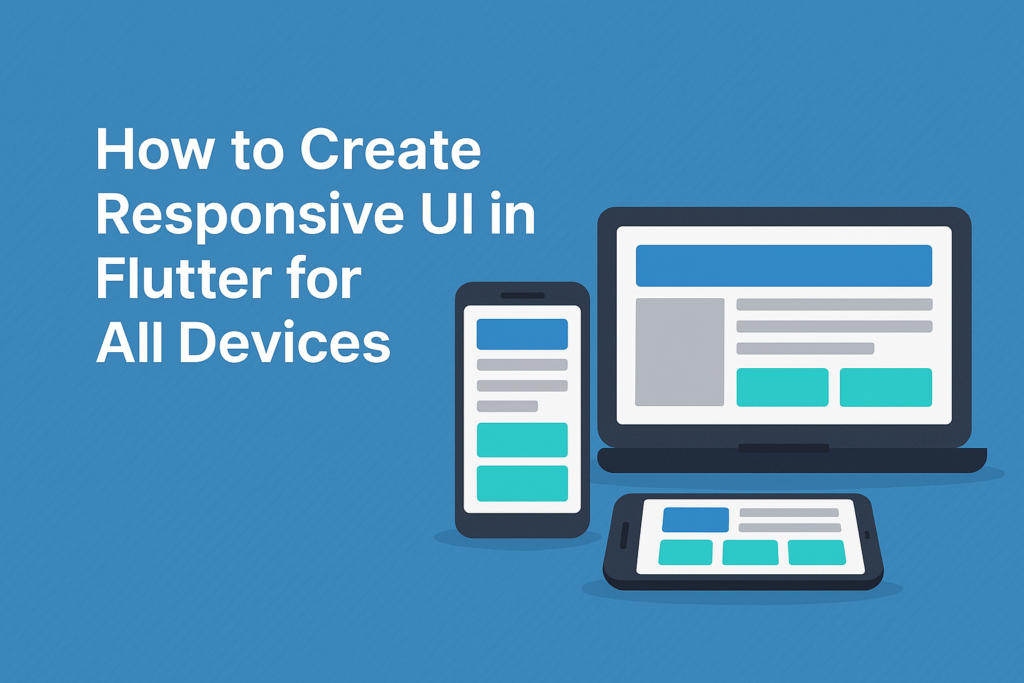
Creating a great app isn’t just about beautiful UI—it’s about building responsive UI in Flutter that works perfectly across phones, tablets, foldables, and desktops. Want to see how the Flutter team approaches this? Check out the official Flutter responsive UI guide.
📱 Why Responsive Design Matters in Flutter
Flutter runs on everything—from Android to iOS, tablets, and even the web. But if your UI isn’t responsive, it’ll:
- Look awkward on tablets or wide screens
- Break when rotated
- Deliver a bad user experience
Responsive UI ensures that your app adapts to any screen size, no matter the device.
🛠️ Flutter Tools for Responsive Design
Flutter provides several built-in widgets and techniques to help you build adaptive UIs:
1. MediaQuery
Use MediaQuery.of(context).size
to get the screen width and height.
double screenWidth = MediaQuery.of(context).size.width;
if (screenWidth < 600) {
// Mobile layout
} else {
// Tablet/Desktop layout
}
Best for: fine-tuned control when you need to adapt based on exact screen size.
2. LayoutBuilder
Gives you access to the parent widget’s constraints—perfect for responsive layouts.
LayoutBuilder(
builder: (context, constraints) {
if (constraints.maxWidth < 600) {
return MobileView();
} else {
return TabletOrDesktopView();
}
},
)
Best for: building flexible components that change layout depending on available space.
3. Flexible
, Expanded
, and Spacer
Use these widgets with Row
and Column
to create flexible UIs that adapt naturally.
Row(
children: [
Expanded(child: Text("Left")),
Spacer(),
Expanded(child: Text("Right")),
],
)
Best for: dynamic sizing within rows/columns.
4. FittedBox
and AspectRatio
Helps maintain element proportions across screens.
AspectRatio(
aspectRatio: 16 / 9,
child: Container(color: Colors.blue),
)
Best for: media-heavy UIs or cards that should maintain shape.
5. Wrap
and GridView
When content should wrap or break across lines (like buttons or cards), use Wrap
or a responsive GridView
.
Wrap(
spacing: 10,
runSpacing: 10,
children: [...],
)
Best for: creating flexible grids or tag systems.
This will show a layout that switches between column and row depending on screen size.
🧠 Pro Tips
- Define your own breakpoints: Mobile (<600), Tablet (600–1024), Desktop (1024+)
- Keep the UX consistent, not just the layout
- Test using Flutter’s built-in Device Preview plugin or run in Chrome and resize
🔚 Conclusion
Responsive UI isn’t just a nice-to-have—it’s essential in Flutter apps that run on all platforms.
Use widgets like LayoutBuilder
, MediaQuery
, Wrap
, and Flex
wisely to build interfaces that scale beautifully.
Pingback: How to Use Custom Fonts and Google Fonts in Flutter - TeachMeIDEA