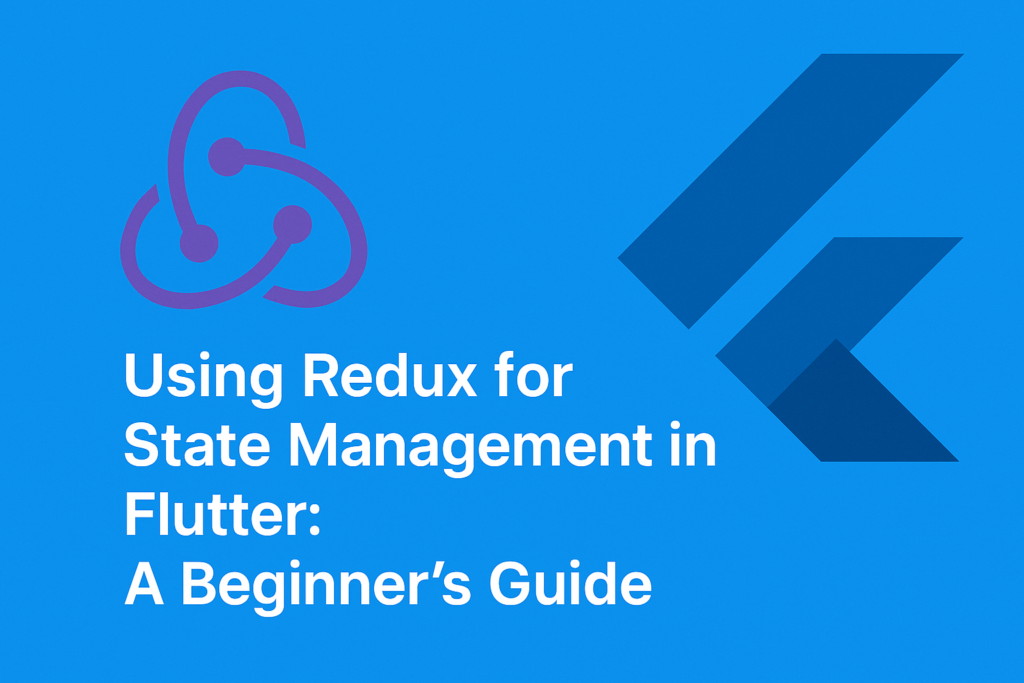
As your Flutter app grows in complexity, managing state becomes more important—and often more challenging. While Flutter has many state management options, Redux remains a popular and powerful choice for developers who want predictable state, centralized control, and a scalable architecture.
In this post, you’ll learn the fundamentals of using Redux in Flutter, how it works, and how to set it up in a simple Flutter app.
What Is Redux in Flutter?
Redux is a state management pattern inspired by Facebook’s Flux architecture. It’s widely known in the web world, especially in React, and applies well to Flutter too.
It operates on a few simple principles:
- There’s a single source of truth: your app’s state lives in one place.
- State is immutable: instead of modifying it directly, you dispatch actions.
- Reducers decide how the state changes based on actions.
Because of this structure, Redux makes your app’s data flow easier to trace and debug.
Why Use Redux in Flutter?
Flutter developers use Redux to manage complex, shared app state in a predictable way. In addition, it offers great scalability and a clear separation of concerns.
Key benefits:
- All state is stored centrally in one place
- Changes happen through dispatched actions
- It’s easier to debug, especially with tools like Redux DevTools
- Works great in large apps with multiple interconnected widgets
Step-by-Step Setup in Flutter
Let’s walk through setting up Redux in a Flutter app with a simple counter example.
Step 1: Add Dependencies for Redux in Flutter
dependencies:
flutter_redux: ^0.10.0
redux: ^5.0.0
You can check the full documentation for the flutter_redux package here.
Step 2: Define App State
class AppState {
final int counter;
AppState({required this.counter});
AppState.initial() : counter = 0;
}
Step 3: Define Actions
class IncrementAction {}
class DecrementAction {}
Step 4: Create a Reducer in Redux in Flutter
AppState counterReducer(AppState state, dynamic action) {
if (action is IncrementAction) {
return AppState(counter: state.counter + 1);
} else if (action is DecrementAction) {
return AppState(counter: state.counter - 1);
}
return state;
}
Step 5: Initialize Redux in main.dart
final store = Store<AppState>(
counterReducer,
initialState: AppState.initial(),
);
void main() {
runApp(MyApp(store: store));
}
Step 6: Connect the UI
class MyApp extends StatelessWidget {
final Store<AppState> store;
const MyApp({required this.store});
@override
Widget build(BuildContext context) {
return StoreProvider<AppState>(
store: store,
child: MaterialApp(
home: CounterPage(),
),
);
}
}
Step 7: Build the Counter UI
class CounterPage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return StoreConnector<AppState, int>(
converter: (store) => store.state.counter,
builder: (context, counter) {
return Scaffold(
appBar: AppBar(title: Text('Redux Counter')),
body: Center(child: Text('Count: $counter')),
floatingActionButton: Row(
mainAxisAlignment: MainAxisAlignment.end,
children: [
FloatingActionButton(
onPressed: () => StoreProvider.of<AppState>(context).dispatch(IncrementAction()),
child: Icon(Icons.add),
),
SizedBox(width: 16),
FloatingActionButton(
onPressed: () => StoreProvider.of<AppState>(context).dispatch(DecrementAction()),
child: Icon(Icons.remove),
),
],
),
);
},
);
}
}
Conclusion
Redux provides a reliable and scalable solution for managing state in Flutter apps. While it introduces more structure and some boilerplate, the long-term benefits in larger apps are worth it. With predictable data flow and centralized control, debugging becomes easier and development more maintainable.
If you’re looking for consistency and control in your state management, Redux is a solid place to start.
Want to explore how Redux compares to other options? Read our breakdown of Provider, Riverpod, and BLoC.