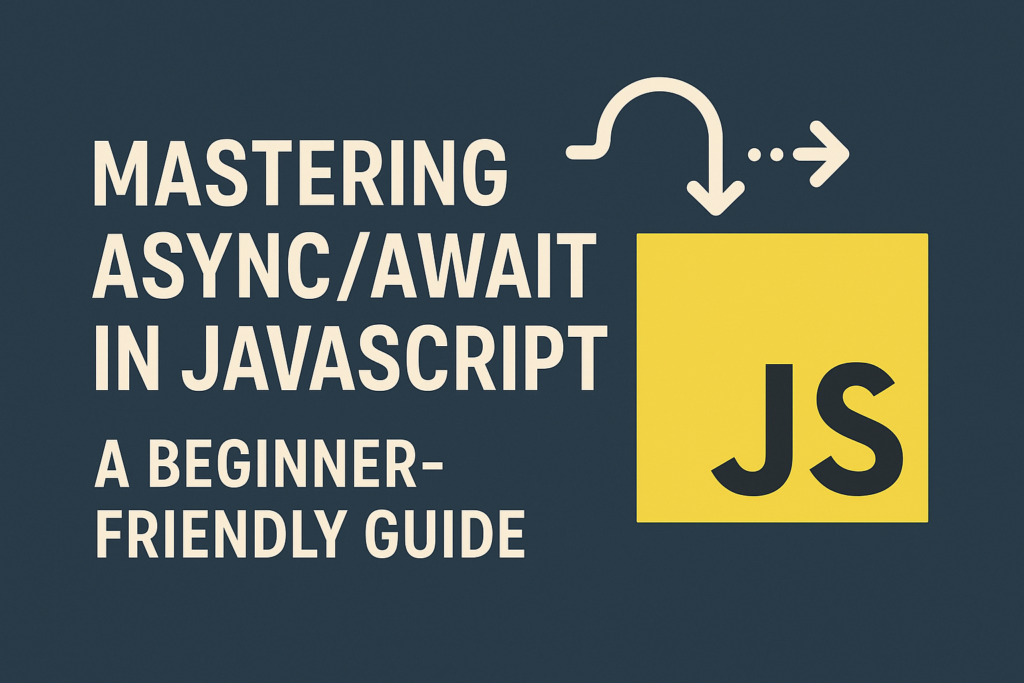
Asynchronous programming is essential in modern JavaScript. Whether you’re fetching data from an API, reading a file, or waiting for user interaction, async code lets your app stay responsive. One of the most beginner-friendly and powerful ways to write async code is with async
/await
.
In this guide, you’ll learn how async
/await
works, how to use it properly, and how it compares to older methods like callbacks and promises.
🧠 What Is Asynchronous Programming?
In JavaScript, tasks like API calls don’t happen instantly. Asynchronous programming lets your code wait for results without freezing the UI. Instead of blocking other operations, async code tells JavaScript to “do this in the background and let me know when it’s done.”
⏳ From Callbacks to Promises to Async/Await
Before async
/await
, developers used:
- Callbacks: Function inside a function → hard to read (“callback hell”)
- Promises: Cleaner syntax, but still involves
.then()
chains
Example using a Promise:
fetch('/api/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(error));
✅ Using Async/Await (The Modern Way)
The async
keyword lets you write functions that return promises, and await
pauses execution until a promise resolves.
async function fetchData() {
try {
const response = await fetch('/api/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error:', error);
}
}
Much cleaner, right?
🧩 Key Rules for Using Async/Await
- You can only use
await
inside anasync
function await
works with promises- Use
try/catch
to handle errors async
functions always return a promise
💡 Practical Use Case: API Calls
const getUser = async (userId) => {
const res = await fetch(`https://jsonplaceholder.typicode.com/users/${userId}`);
return await res.json();
};
getUser(1).then(user => console.log(user));
Perfect for apps fetching data on load, such as user profiles or blog posts.
⚠️ Common Mistakes to Avoid
- ❌ Forgetting to use
await
with an async function - ❌ Not wrapping
await
calls intry/catch
- ❌ Using
await
inside loops without care (can be slow; preferPromise.all
if possible)
🔗 Useful Resources
✅ Final Thoughts
async
/await
is one of the best things to happen to JavaScript. It helps you write clean, readable, and modern asynchronous code. Once you get used to it, you’ll never want to go back to .then()
chains or messy callbacks again.
Start experimenting with it in your projects — especially when working with APIs, files, or any background tasks.
Pingback: TypeScript vs JavaScript: Key Differences You Should Know - TeachMeIDEA