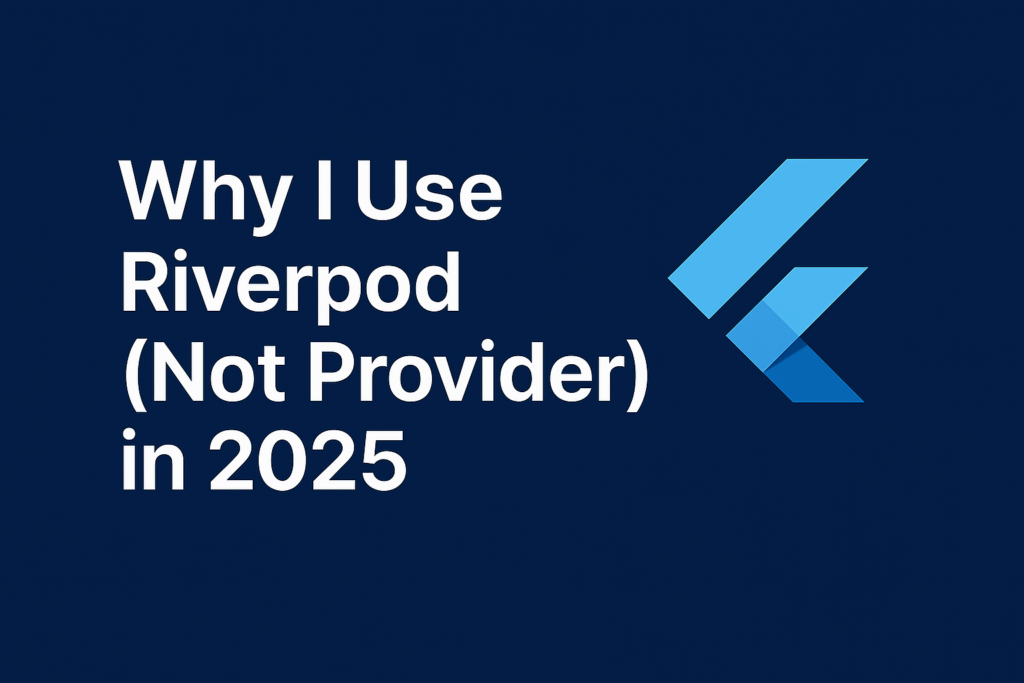
Flutter has no shortage of state management solutions, but for years, Provider was the go-to. It was simple, effective, and easy to integrate. But in 2025, my default choice is Riverpod—and I haven’t looked back.
In this post, I’ll break down why I prefer Riverpod over Provider, how it solves real development pain points, and why it’s the future of state management in Flutter.
🔁 Provider Was Great… Until It Wasn’t
Let’s give Provider credit—it taught many of us about Flutter state management. It was easy to use, reactive, and supported scoped state well.
But as my projects grew, Provider started showing limitations:
- Tight coupling to the widget tree
- Difficult to reuse logic across features
- Harder to test outside the UI
- Boilerplate for complex patterns
- Unexpected rebuilds and lifecycle confusion
🧠 Why I Switched to Riverpod
Riverpod is a complete rewrite of Provider, by the same author (Remi Rousselet). It keeps the good parts of Provider—but fixes the frustrating stuff.
Here’s what made me switch:
✅ 1. No More Widget-Tree Ties
In Provider, you often had to wrap widgets in MultiProvider
, which can become a mess. In Riverpod, providers live outside the widget tree. This means:
- Better separation of concerns
- No need to refactor widgets just to inject state
- Logic can live independently and cleanly
✅ 2. Testability Without the Hassle
I can test my Riverpod providers without needing a WidgetTester
or running Flutter-specific code. Just create a ProviderContainer
and write pure Dart tests.
final container = ProviderContainer();
final value = container.read(myProvider);
Simple, fast, and UI-agnostic.
✅ 3. Better Async State Handling
With AsyncValue and AsyncNotifier, Riverpod makes handling loading, success, and error states a breeze. I no longer have to write extra boilerplate or create flags to track loading manually.
ref.watch(myProvider).when(
loading: () => CircularProgressIndicator(),
data: (data) => Text('$data'),
error: (e, _) => Text('Error: $e'),
);
✅ 4. Scoped Overrides Are a Superpower
Need to override a provider in a test or a specific part of the app? Riverpod makes this extremely clean and safe.
ProviderScope(
overrides: [
myProvider.overrideWithValue(FakeService()),
],
child: MyApp(),
);
This is one of the biggest reasons I now prefer Riverpod in modular or test-driven projects.
✅ 5. Cleaner Architecture
Riverpod naturally encourages feature-based organization. Combined with ref
, watch
, and read
, I can build feature modules that are easy to reason about and scale across large teams.
📈 Why It Matters in 2025
Flutter apps today are more complex than ever—multiple environments, shared logic, dependency injection, test automation, you name it.
Riverpod is built for scalability, and in 2025, that matters. It gives me:
- Confidence when refactoring
- Clean dependency injection
- Isolated logic that scales
- Faster tests
- Modern dev tools
🧠 Final Thoughts
Provider still works. If you’re building a simple app, there’s nothing wrong with using it. But if you want:
- A better developer experience
- Cleaner testing
- More predictable architecture
- A scalable future
Then Riverpod is the state management tool to bet on in 2025.
And that’s why I use it—every time.